Binary flags with Python
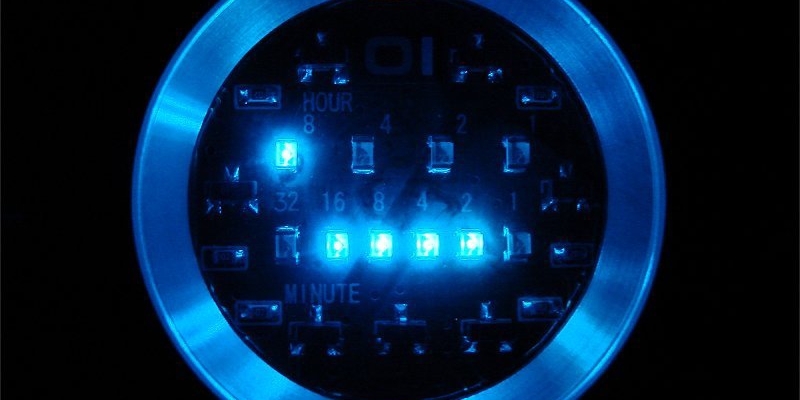
Sometimes you just want to run a few specific sections of your python code for testing. Then a few more. Next, all sections. Now run just one. Or possibly need to run different parts of your code based on the time of day or week.
There are many ways you can accomplish this directly in your python script(s) or by using the python window. But what about passing a runtime argument with a little binary?! This technique is a great way to run different/multiple sections (functions) of your code without the need to modify the python script - binary flags with python. I even use this technique to keep some test functions to call them when needed - and also to run minor variations based on time of day.
The method behind this approach is to use binary (base 2 numbers), but not using a long string of 0’s and 1’s. Rather, converting regular long integers (base 10) into binary in order to determine what sections of code to run.
Setup
You have 7 sections of code broken down into functions. Each section is assigned a value - these values are set to the power of 2 (assign the number on the far right side), examples:
- Section 0 = 2^0 = 1
- Section 1 = 2^1 = 2
- Section 2 = 2^2 = 4
- Section 3 = 2^3 = 8
- Section 4 = 2^4 = 18
- Section 5 = 2^5 = 32
- Section 6 = 2^6 = 64
- and so on…
Usage
Whenever you want to call multiple sections, just add up the values of each section and pass as a runtime argument.
- Run section 3 only: MyScript.py 8
- Run section 4 only: MyScript.py 18
- Run section 1, 3, and 5 (2+8+32): MyScript.py 42
Since you are passing the integer (base 10) number as the argument, we need add a small function to the python script to determine the sections to run. The functions/sections will always be run from lowest to highest (highest to lowest is possible with a small modification to the script).
The code
Using this small function, we can determine which section(s) to run.
def fn_bits(n):
while n:
b = n & (~n+1)
yield b
n ^= b
Putting it all together
Now that we can pass the commands we want to run as a single argument, we need to put it all together. Here is a working sample of binary flags with python.
Based on the full code sample above, here is how we would call different code combinations:
- Section 0 only:
BitFlagFunctions.py 1
- Section 2 and 3 (4+8):
BitFlagFunctions.py 12
- Section 0, 2, and 3 (1+4+8):
BitFlagFunctions.py 13
- Section 1 and 3 (2+8):
BitFlagFunctions.py 10
Summary
This is just one of many ways you can setup binary flags with python. I’ve seen other examples where people use “0100101” to run various sections which also works well. The method described in the above gist is just a way that works well for me, giving me the ability to number each section and run them in ascending order as needed.
If you found my writing entertaining or useful and want to say thanks, you can always buy me a coffee.